C++ Functions
In this tutorial, we will learn about the C++ function and function expressions with the help of examples.
Functions
- A function is a block of code which is used to performs a specific task.
- Functions are used to provide modularity & code reusability to a program.
- The Function is also known as procedure or subroutine in other programming languages.
- Every C++ program has atleast one function, which is
main()
. - Dividing a complex problem into smaller chunks makes our program easy to understand and reusable.
For example : we need to create a program to create a Square and color it. We can create two functions to solve this problem:
- First function : this function is used to draw the Square
- Second function : this function is used to color the Square
Advantages of Functions
- It makes the program clear and easy to underatand.
- Single Functions can be tested easily for errors.
- It saves time from typing teh same function again and again.
- It helps to modify the program easily without changing the structure of a program.
- Function can be called multiple times as per requirement.
Types of Function
There are two types of function:
- Standard Library Functions: are the functions which are Predefined in C++.
- User-defined Function: are the functions which are Created by users.
In this tutorial, we will focus mostly on user-defined functions.
C++ User-defined Function
- User-Defined function are those functions which are defined by user.
- It allows performing the additional functions besides the in-build functions.
- A user-defined function groups code to perform a specific task and that group of code is given a name (identifier).
- When the function is invoked from any part of the program, it all executes the codes defined in the body of the function.
C++ Function Declaration
- In C++, the function declaration tells the compiler about a function name and how to call the function.
- A function declaration also tells about the number of parameters function takes, data-types of the parameters and the return type of the function.
- Putting parameter names in function declaration is Optional in the function declaration, but it is necessary to put them in the definition.
The syntax to declare a function is:
returnType functionName (parameter1, parameter2,...) {
// function body
}
Here's an example of a function declaration.
// function declaration
void hey() {
cout << "Hello World!";
}
Here,
- the name of the function is
hey()
- the return type of the function is
void
- the empty parentheses mean it doesn't have any parameters
- the function body is written inside
{}
Note: We will learn about
returnType
and parameters
later in this tutorial.
Calling a Function
- when a program calls a function, program control is transferred to the called function.
- A called function performs defined task and when it's return statement is executed, it returns program control back to main program.
- To call a function, we need to pass the requirement parameters along with function name, and if function returns a value, then we can store returned value.
for example :
In the above program, we have declared a function named hey()
.
To use the hey()
function, we need to call it.
Here's how we can call the above hey()
function.
int main() {
// calling a function
hey();
}
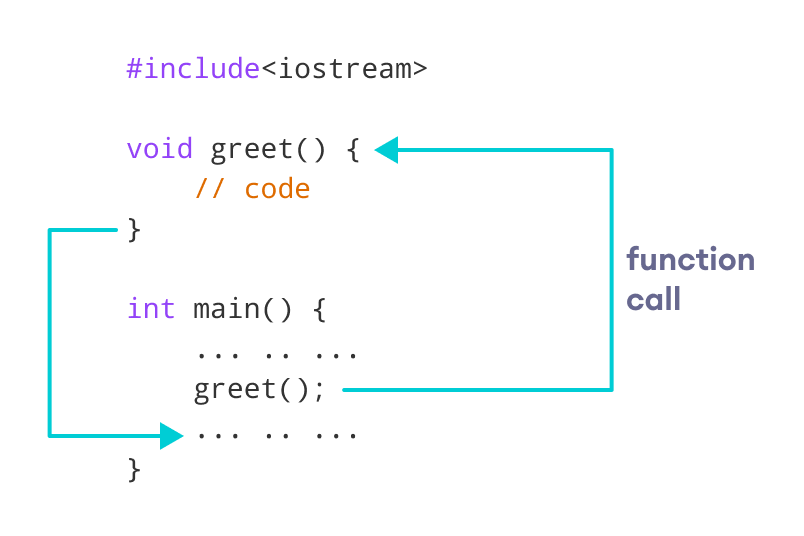
Example 1: C++ Program to Display Hello world using function
// This program to check if you are 18 or not using goto statement.
# include <iostream>
using namespace std;
// declaring a function
void hey() {
cout << "Hello world!";
}
int main() {
// calling the function
hey();
return 0;
}
Output
Hello world!
Function Parameters
- Parameters are variables to hold values of arguments passed while function is called.
- A function may or may-not contain parameter list.
- There are two types of parameters actual and formal parameters that are passed in a function.
- The actual parameters are passed in a function like a = 20, b = 10.
- And the formal parameters are those parameters which are received by the function like int c and int d.
As mentioned above, a function can be declared with parameters (arguments). A parameter is a value that is passed when declaring a function.
For example, let us consider the function below:
void printN(int a) {
cout << a;
}
Here, the int
variable a is the function parameter.
We pass a value to the function parameter while calling the function.
int main() {
int a = 7;
// calling the function
// a is passed to the function as argument
printN(a);
return 0;
}
Example 2: C++ program to print integer and floting value using Function with Parameters
// program to print integer and floting value.
#include <iostream>
using namespace std;
// print a number
void Number(int num1, float num2) {
cout << "The integer number is " << num1 << endl;
cout << "The decimal number is " << num2;
}
int main() {
int a = 2;
double b = 2.5;
// calling the function
Number(a, b);
return 0;
}
Output
The integer number is 2 The decimal number is 2.5
Working of above program
we have used a function that has one int
parameter and one double
parameter.
We then pass a and b as arguments. These values are stored by the function parameters num1 and num2 respectively.
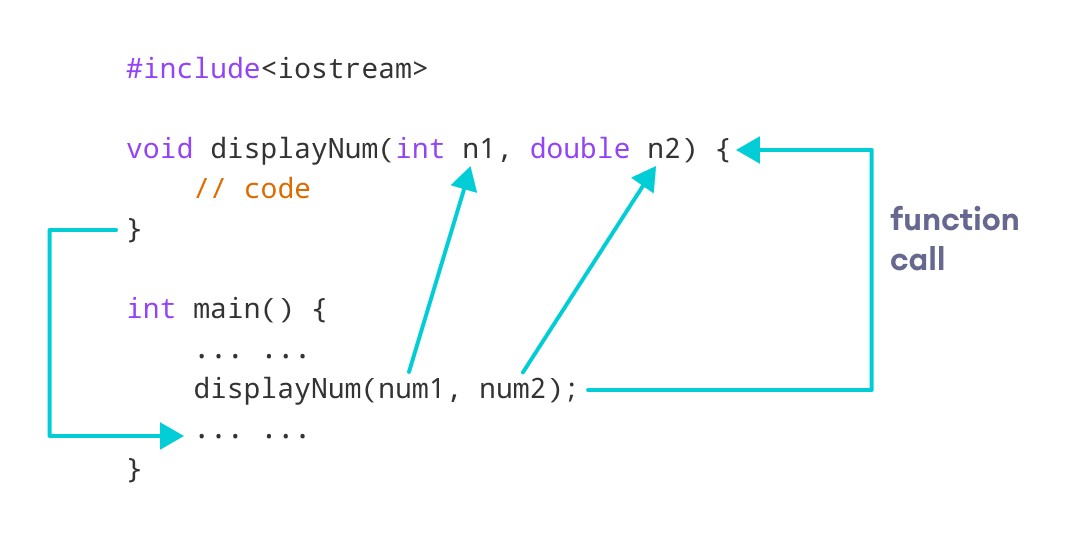
Note: The type of the arguments passed while calling the function must match with the corresponding parameters defined in the function declaration.
Return Statement
In the above programs, we have used void in the function declaration. For example,
void Number() {
// statement
}
- This means the function is not returning any value.
- It's also possible to return a value from a function.
- For this, we need to specify the
returnType
of the function during function declaration. - Then, the
return
statement can be used to return a value from a function.
For example,
int add (int a, int b) {
return (a + b);
}
Here, we have the data type int
instead of void
. This means that the function returns an int
value.
The code return (a + b);
returns the sum of the two parameters as the function value.
The return
statement denotes that the function has ended. Any code after return
inside the function is not executed.
Example 3: C++ program to add Two Numbers using Function
// program to add two numbers using a function
#include <iostream>
using namespace std;
// declaring a function
int add(int a, int b) {
return (a + b);
}
int main() {
int sum;
// calling the function and storing the returned value in sum
sum = add(10, 50);
cout << "10 + 50 = " << sum << endl;
return 0;
}
Output
10 + 50 = 60
In the above program, the add()
function is used to find the sum of two numbers.
We pass two int
literals 10
and 50
while calling the function.
We store the returned value of the function in the variable sum, and then we print it.
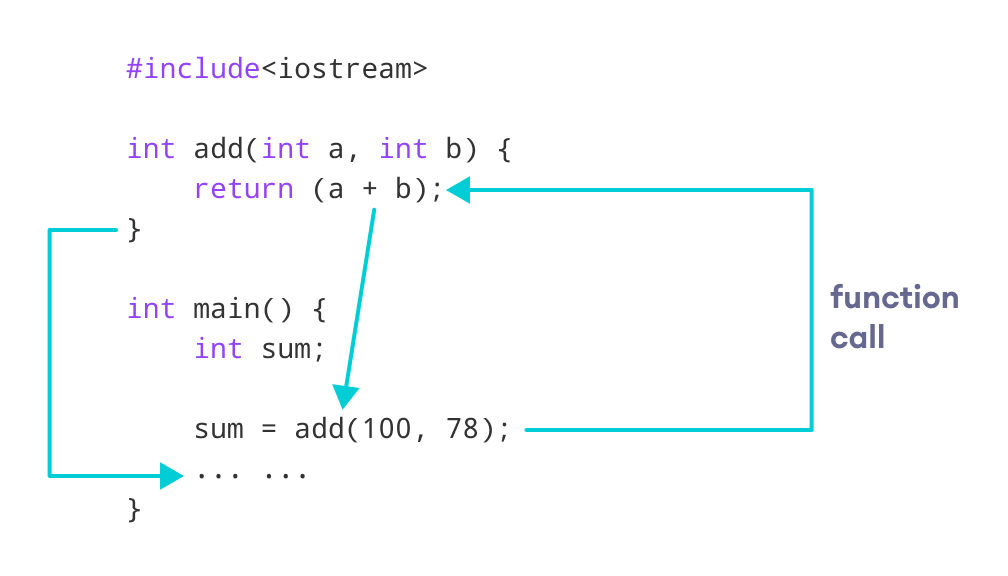
Notice that sum is a variable of int
type. This is
because the return value of add()
is of int
type.
Function Prototype
- In C++, the code of function declaration should be before the function call.
- However, if we want to define a function after the function call, we need to use the function prototype.
- It tells the return type of the data that the function will return.
- It tells the number of arguments passed to the function.
- It tells the data types of the each of the passed arguments
- It also tells the order in which the arguments are passed to the function.
For example:-
// function prototype
void add(int, int);
int main() {
// calling the function before declaration.
add(2, 4);
return 0;
}
// function definition
void add(int a, int b) {
cout << (a + b);
return 0;
}
Output
6
This provides the compiler with information about the function name and its parameters. That's why we can use the code to call a function before the function has been defined.
In the above code, the function prototype is:
void add(int, int);
The syntax of a function prototype is:
returnType functionName(dataType1, dataType2, ...);
Example 4: Add two numbers using Function Prototype
// using function definition after main() function
// function prototype is declared before main()
#include <iostream>
using namespace std;
// function prototype
int add(int, int);
int main() {
int sum;
// calling the function and storing
// the returned value in sum
sum = add(10, 50);
cout << "10 + 50 = " << sum << endl;
return 0;
}
// function definition
int add(int a, int b) {
return (a + b);
}
Output
10 + 50 = 60
The above program is almost similar to Example 3. The only difference is that here, the function is defined after the function call.
That's why we have used a function prototype in this example.
Advantages of Using User-Defined Functions
- Reduction in size - This avoids writing of same code again and again reducing program size.
- Reducing complexity - Functions make the program easier as each small task is divided into a function. or user defined functions.
- Readability - Functions increase readability.
- Easy to Debug and Maintain - During debugging it is very easy to locate and isolate faulty functions. It is also easy to maintain program that uses user-defined functions.
- Code Reusability - Functions make the code reusable. We can declare them once and use them multiple times.
C++ Library Functions
- Library functions are the built-in functions in C++ programming.
- Library functions are those type of functions which are already defined, compiled and stored in different header files of standard C++ library.
- Users can use library functions by invoking the functions directly; they don't need to write the functions themselves.
For example :
Some common library functions in C++ are sqrt()
,
abs()
, isdigit()
, etc.
In order to use mathematical functions such as sqrt()
and abs()
, we need to include the header file cmath
.
Example 5: Program to Find the Square Root of a Number
#include <iostream>
// declaring "cmath" header file to use mathematical functions
#include <cmath>
using namespace std;
int main() {
int number, squareRoot;
number = 4;
// sqrt() is a library function to calculate the square root
squareRoot = sqrt(number);
cout << "Square root of " << number << " = " << squareRoot;
return 0;
}
Output
Square root of 4 = 2
In this program, the sqrt()
library function is used to calculate the square root of a number.
The function declaration of sqrt()
is defined in the
cmath
header file. That's why we need to use the code
#include <cmath>
to use the sqrt()
function.
Difference b/w User-Defined and Library functions
User-Defined Function | Library Functions |
---|---|
These functions are not predifined in the compiler. | These functions are predifined in the compiler of C++ language. |
These functions are Created by user as per their own requirement. | These functions are not created by user as their own requirement. |
User-defined functions are not stored in library file. | Library functions are stored i n special library file. |
Execution of the program begins from teh user-define function. | Execution of the program does not begin from the library function. |
There is no such kind of requirement to add the particular library. | If user want to use library function, It's mandatory to use particular library of that function in header file of the program. |
Next Tutorial
We hope that this tutorial helped you develop better understanding of the concept of Functions in C++.
Keep Learning : )
In the next tutorial, you'll learn about C++ user-defined function types
.