C++ Continue Statement
In this tutorial, we will learn about Continue Statement and its working in loops with the help of examples.
Continue Statement
- Continue statement is a loop Control statement used inside the loop.
- Continue is also a loop control statement just like the break statement.
- Continue statement is opposite to that of break statement, instead of terminating the loop, it forces to execute the next iteration of the loop.
- In computer programming, the continue statement is used to skip the current iteration of the loop and the control of the program goes to the next iteration.
The syntax of the continue
statement is:
continue;
To understand Continue
statement, you should have the knowledge of the following C++ programming topics:
Working of C++ continue Statement
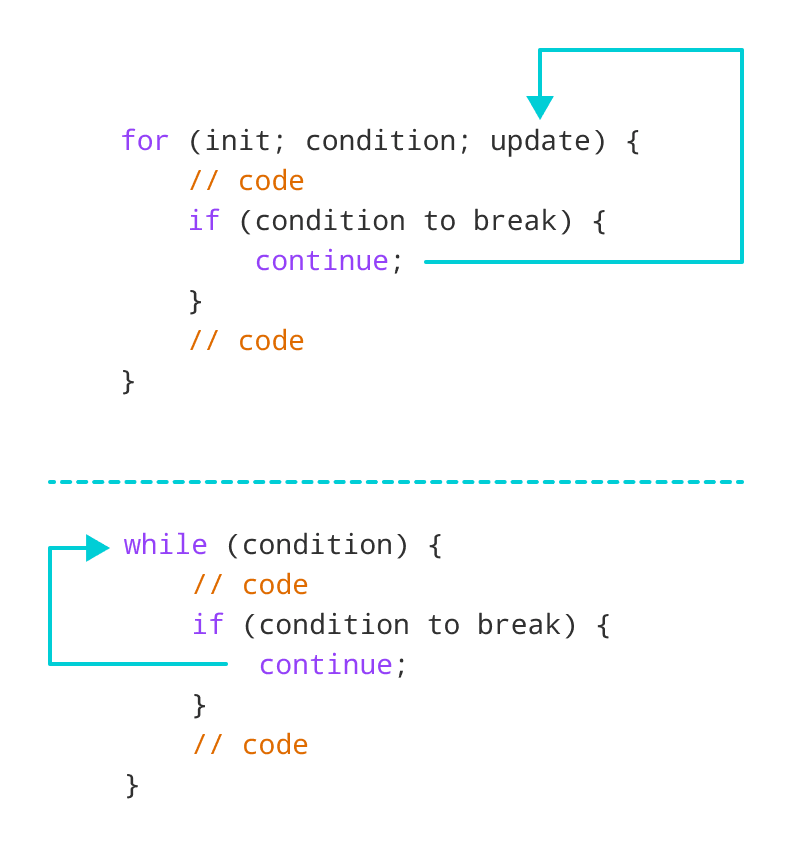
Example 1: continue with for loop
// program to explain the use of continue statement inside for loop
#include <iostream>
using namespace std;
// loop to print numbers 1 to 10
int main() {
for (int i = 1; i <= 10; i++) {
// if i is equal to 4 , continue to next iteration without printing 4.
if (i == 4) {
continue;
}
else{
// otherwise print the value of i.
cout << i << " ";
}
}
return 0;
}
Output
1 2 3 5 6 7 8 9 10
In the above program, we have used the the for
loop to print the
value of i in each iteration. Here, notice the code,
Note: The break
statement is usually used with decision-making statements.
if (i == 4) {
continue;
}
This means
- When i is equal to
4
, thecontinue
statement skips the current iteration and starts the next iteration - Then, i becomes
5
, and thecondition
is evaluated again. - Hence,
5
and6
are printed in the next two iterations. - Loop prints the value of i until it became
11
and condition becomes fales. Then, the loop terminates.
Note:
1) The continue
statement is almost always used with decision-making statements.
2) The break
statement terminates the loop entirely. However, the continue
statement only skips the current iteration.
Example 2: C++ continue with while loop
In a while
loop, continue
skips the current iteration and control flow of the program jumps back to the while
condition.
// program to find the sum of positive numbers
// if the user enters a negative numbers, Continue statement skips that number
#include <iostream>
using namespace std;
int main() {
int sum = 0;
int num = 0;
while ( num >=0) {
// take input from the user
cout << "Enter a number: ";
cin >> num;
// continue condition
if (num > 30) {
cout << "The number is greater than 50 and won't be calculated." << endl;
num = 0; // the value of number is made 0 again
continue;
}
// add all the positive integers
sum += num;
}
// display the sum
cout << "The sum of positive numbers: " << sum << endl;
return 0;
}
Output
Enter a number: 24 Enter a number: 16 Enter a number: 22 Enter a number: 32 The number is greater than 30 and won't be calculated. Enter a number: -4 The sum of positive numbers: 58
In the above program, the user enters a number. The while
loop is used to print the total sum of positive numbers entered by the user, as long as the numbers entered are not greater than 30
.
Notice the use of the continue
statement.
if (num > 30){
continue;
}
- When the user enters a number greater than
30
, thecontinue
statement skips the current iteration. Then the control flow of the program goes to thecondition
ofwhile
loop. - When the user enters a number less than
0
, the loop terminates.
Example 3: Continue statement with do-while loop
// C++ Program to print Continue statement inside do-while loop
#include <iostream>
using namespace std;
int main() {
// Initialization expression
int i = 1;
// do...while loop execution
do {
cout << "Value of i: " << i << endl ;
i++;
if (i == 4){
i++;
// it skips the value
continue;
}
}while (i <= 8);
return 0;
}
Output
Value of i: 1 Value of i: 2 Value of i: 3 Value of i: 5 Value of i: 6 Value of i: 7 Value of i: 8
In the above program, we have used the the do-while
loop to print the
value of i in each iteration. Here, notice the code,
if (i == 4) {
continue;
}
This means
- When i is equal to
4
, thecontinue
statement skips the current iteration and starts the next iteration - Then, i becomes
5
, and thecondition
is evaluated again. - Hence,
5
and6
are printed in the next two iterations. - Loop prints the value of i until it became
9
and condition becomes fales. Then, the loop terminates.
Hence, the value of i == 4 is never displayed in the output.
Example 4: continue with Nested loop
// using continue statement inside
// nested for loop
#include <iostream>
using namespace std;
int main() {
// nested for loops
// Outer loop
for (int i = 1; i <= 2; i++) {
//Inner loop
for (int j = 1; j <= 3; j++) {
if (j == 2) {
continue;
}
cout << "i = " << i << ", j = " << j << endl;
}
}
return 0;
}
i = 1, j = 1 i = 1, j = 3 i = 2, j = 1 i = 2, j = 3
In the above program, when the continue
statement executes, it skips the current iteration in the inner loop. And the control of the program moves to the update expression of the inner loop.
Hence, the value of j = 2 is never displayed in the output.
Next Tutorial
We hope that this tutorial helped you develop better understanding of the concept of Continue Statement in C++.
Keep Learning : )
In the next tutorial, you'll learn about C++ Switch Case Statement
.