C++ break Statement
In this tutorial, we will learn about the break statement and its working in loops with the help of examples.
Break Statement
- The break is a loop control statement which is used to terminate the loop.
- It is used to stop the current execution and proceed with the next one.
- Basically break statements are used in the situations when we are not sure about the actual number of iterations for the loop or we want to terminate the loop based on some condition.
- When the break statement is called by a compiler, it immediately stops the execution of the loop and transfers the control outside the loop and executes the other statements.
In C++, the break
statement terminates the loop when it is encountered.
The syntax of the break
statement is:
break;
To understand break
statement, you should have the knowledge of the following C++ programming topics:
Working of C++ break Statement
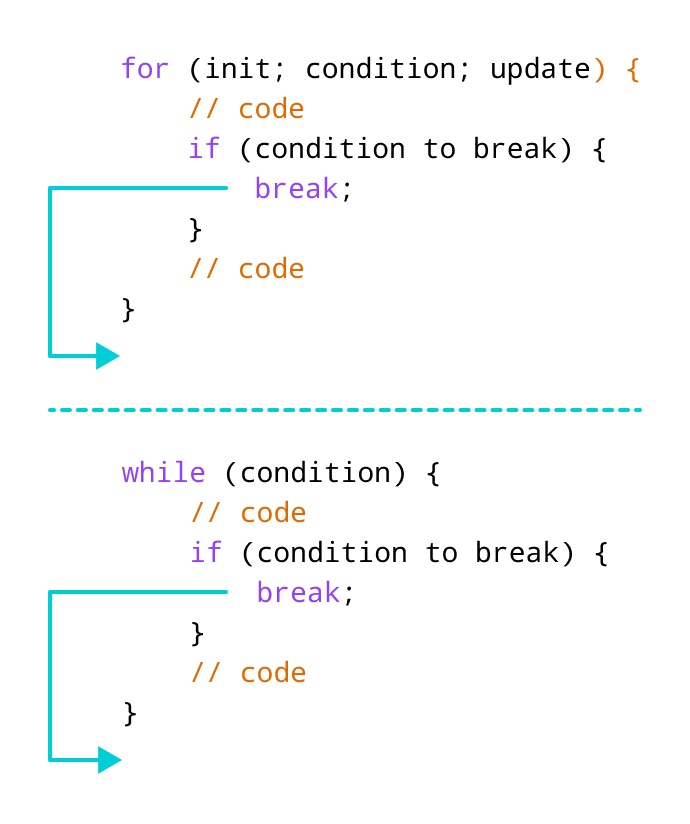
Example 1: break statement with for loop
// program to use break statement inside for loop
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 8; i++) {
// break condition
if (i == 5) {
break;
}
cout << i << endl;
}
return 0;
}
Output
1 2 3 4
This means, when i is equal to 5, the break
statement terminates the loop. Hence, the output doesn't include values greater than or equal to 5.
Note: The break
statement is usually used with decision-making statements.
Example 2: C++ break statement with while loop
// program to find the sum of positive numbers
// if the user enters a negative numbers, break ends the loop
#include <iostream>
using namespace std;
int main() {
int num;
int sum = 0;
while (true) {
// take input from the user
cout << "Enter a number: ";
cin >> num;
// break condition
if (num < 0) {
break;
}
// add all the positive integers
sum += num;
}
// display the sum
cout << "The sum of positive numbers: " << sum << endl;
return 0;
}
Output
Enter a number: 3 Enter a number: 4 Enter a number: 5 Enter a number: -4 The sum of positive numbers: 12
In the above program, the user enters a number. The while
loop is used to print the total sum of numbers entered by the user. Here, notice the code,
if(num < 0) {
break;
}
This means, when the user enters a negative number, the break
statement terminates the loop and codes outside the loop are executed.
The while
loop continues until the user enters a negative number.
Example 3: break statement with Nested loop
When break
is used with nested loops, break
statement terminates the inner loop. For example,
// using break statement inside nested for loop
#include <iostream>
using namespace std;
int main() {
int num;
int sum = 0;
// nested for loops
// first loop
for (int i = 1; i <= 3; i++) {
// second loop
for (int j = 1; j <= 2; j++) {
if (i == 2) {
break;
}
cout << "i = " << i << ", j = " << j << endl;
}
}
return 0;
}
Output
i = 1, j = 1 i = 1, j = 2 i = 3, j = 1 i = 3, j = 2
In the above program, the break
statement is executed when
i == 2
. It terminates the inner loop, and the control flow
of the program moves to the outer loop.
Hence, the value of i = 2 is never displayed in the output.
Example 4: break statement with do-while loop
// C++ Program to print break statement inside do-while loop
#include <iostream>
using namespace std;
int main() {
// Initialization expression
int i = 5;
// do...while loop execution
do {
cout << "Value of i: " << i << endl ;
i = i + 1;
if (i > 10){
// terminate the loop
break;
}
}while (i <= 12);
return 0;
}
Value of i: 5 Value of i: 6 Value of i: 7 Value of i: 8 Value of i: 9 Value of i: 10
In the above program, the break
statement is executed when
i > 10
. It terminates the inner loop, and the control flow
of the program moves to the outer loop.
Hence, the value of i > 2 is never displayed in the output.
The break
statement is also used with the switch
statement. To learn more, visit C++ switch statement.
Next Tutorial
We hope that this tutorial helped you develop better understanding of the concept of Break Statement in C++.
Keep Learning : )
In the next tutorial, you'll learn about C++ Continue Statement
.